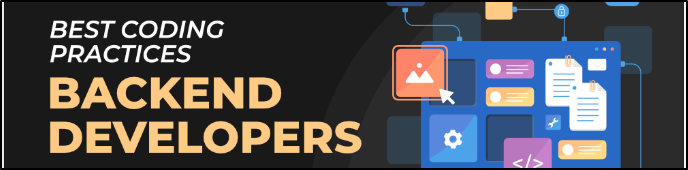
Explore the intricacies of our backend code design as we delve into the architecture, services, and key components shaping the foundation of our system. Join us for a focused discussion on the elegance, scalability, and efficiency embedded in our backend solutions, aimed at delivering optimal performance and maintainability.
- Controller:
- API Endpoints:
/login
,/logout
,/user/{id}
- HTTP Methods:
POST
(login),POST
(logout),GET
(retrieve user details) - Input Validation: Validate login credentials, handle session tokens securely.
- Request/Response Format: JSON format for login/logout requests, user details response.
- Error Handling: Return 401 for authentication failure, 404 for non-existing users.
- API Endpoints:
- Service:
- Business Logic: User authentication, authorization checks.
- External Services: Integration with LDAP for enterprise user validation.
- Caching: Cache user roles for faster authorization checks.
- Transaction Management: Ensure atomic updates during user login/logout.
- Database/Table Design:
- Schema:
User
table with fields likeid
,username
,password_hash
,roles
. - Indexes: Index on
username
for efficient user lookup. - Security: Hash and salt passwords, implement secure password policies.
- Schema:
- Exception Handling:
- Scenarios: Handle exceptions for database errors, authentication failures.
- Logging: Log authentication events, failed login attempts.
- Custom Exceptions: Create custom exceptions like
InvalidCredentialsException
. - Consistency: Maintain consistent error response format across endpoints.
- Logging:
- Log Levels: Use INFO for login/logout events, ERROR for authentication failures.
- Formats: Log in a structured format with timestamp, event type, and user information.
- Centralized Logging: Integrate with centralized logging for monitoring.
- Security:
- Authentication: Use JWT tokens for secure session management.
- Authorization: Implement role-based access control for resource permissions.
- Encryption: Encrypt sensitive data in transit and at rest.
- Secure Communication: Use HTTPS for secure data transmission.
- Testing:
- Unit Testing: Test service methods for login, logout, and authorization.
- Integration Testing: Validate API endpoints for different authentication scenarios.
- Environments: Have separate environments for development, staging, and production.
- Continuous Integration: Automate testing and integrate with CI/CD pipelines.
- Documentation:
- API Documentation: Document endpoints, expected request/response formats.
- Database Documentation: Outline user table schema, indexing, and relationships.
- Internal Documentation: Include code comments for key functions and logic.
- Versioning: Clearly document API versioning and changes.
- Performance:
- Bottlenecks: Identify potential bottlenecks during authentication and authorization.
- Scaling Strategies: Consider horizontal scaling for increased user loads.
- Monitoring: Use performance monitoring tools to track response times.
- Scalability:
- Architecture: Design for scalability with microservices in mind.
- Load Balancing: Implement load balancing to distribute authentication requests.
- Caching: Use a distributed caching system for improved performance.
In conclusion, a well-designed backend code is the backbone of a robust and scalable application. By adhering to best practices, modularizing code, implementing effective error handling, and incorporating thorough testing, developers can ensure maintainability, extensibility, and a smooth user experience. Prioritizing clean and efficient design not only facilitates collaboration within development teams but also lays the foundation for future enhancements and optimizations. Ultimately, thoughtful backend code design is key to building resilient and high-performing software systems.