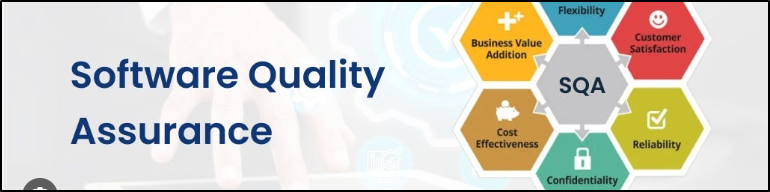
Delivering software code with good quality is crucial for the success of any software development project. It ensures that the software is reliable, maintainable, and meets the requirements of the users. Here’s a step-by-step guide to help you achieve this:
- Requirement Analysis:
- Understand and document the software requirements. This should include functional and non-functional requirements.
- Design:
- Create a detailed design of the software. Use UML diagrams, flowcharts, and other tools to map out the structure and components of your software.
- Coding Standards and Guidelines:
- Define and follow coding standards and guidelines. This ensures that all code is consistent and readable. Tools like linters can help enforce these standards.
- Version Control:
- Use a version control system like Git to track changes to your code. This allows you to collaborate with others, revert to previous versions, and maintain a history of your codebase.
- Code Reviews:
- Implement a code review process. Have peers review your code for quality, adherence to coding standards, and potential issues. Review their code as well.
- Testing:
- Unit Testing:
- Write unit tests for individual components of your code, such as functions or methods. These tests ensure that each piece of code works as intended in isolation.
- Example: For a function that calculates the sum of two numbers, you’d write a unit test to verify that it returns the correct result for different inputs.
- Integration Testing:
- Test how different components of your software interact with each other. Focus on ensuring that the integration points work smoothly.
- Example: In your task management app, you’d test the integration between user authentication and task assignment to confirm that a user can assign a task to another user seamlessly.
- System Testing:
- Test the entire software system as a whole. This helps uncover issues that may not be apparent in unit or integration testing but might emerge due to the combined behavior of all components.
- Example: In your task management app, a system test would involve creating tasks, assigning them, and checking if notifications work correctly.
- User Acceptance Testing (UAT):
- Engage real users to evaluate the software from an end-user perspective. This type of testing ensures that the software meets their expectations and is user-friendly.
- Example: Invite a group of users to your task management app, ask them to perform common tasks, and gather feedback on their experiences.
- Automated Testing:
- Implement automated testing to save time and ensure consistency. Use testing frameworks such as JUnit, Selenium, or PyTest to automate test cases.
- Example: In your task management app, you might use Selenium to automate the testing of user registration and login processes.
- Regression Testing:
- After making changes or adding new features, run regression tests to ensure that existing functionality hasn’t been broken.
- Example: When you add a new feature like task prioritization to your app, you’d re-run tests for existing features to check if they still work as expected.
- Performance Testing:
- Test the software’s performance under different scenarios, such as load testing to evaluate how the system performs under heavy user traffic.
- Example: Simulate 1,000 users concurrently using your task management app and measure its response time and resource utilization.
- Security Testing:
- Identify and mitigate vulnerabilities by conducting security testing. This includes penetration testing to find weaknesses in your software’s security measures.
- Example: Run a penetration test on your task management app to find potential security vulnerabilities, such as weak authentication or data exposure.
- Usability Testing:
- Evaluate the software’s user interface and overall user experience. Usability tests help ensure that the software is user-friendly and intuitive.
- Example: Conduct usability testing by having users perform common tasks in your task management app while providing feedback on the interface design and ease of use.
- Exploratory Testing:
- Allow testers to explore the software freely and interact with it as real users would. This approach helps uncover unexpected issues.
- Example: Give testers access to your task management app without specific instructions and encourage them to explore and perform tasks while reporting any issues they encounter.
- Accessibility Testing:
- Verify that the software is accessible to individuals with disabilities. This testing ensures compliance with accessibility standards, such as WCAG (Web Content Accessibility Guidelines).
- Example: Use screen readers to test the accessibility of your task management app for visually impaired users.
- Localization and Internationalization Testing:
- If your software is intended for a global audience, test it with different languages, regions, and character sets to ensure it functions correctly in various cultural contexts.
- Example: Test your task management app with different languages, currencies, and date formats to confirm that it adapts appropriately to regional settings.
- Compatibility Testing:
- Verify that the software works correctly on various devices, browsers, and operating systems. This is especially important for web applications.
- Example: Test your task management app on different web browsers (e.g., Chrome, Firefox, Edge) and various devices (desktop, mobile) to ensure compatibility.
- User Feedback and Bug Reporting:
- Encourage users to provide feedback and report bugs they encounter during testing. This continuous feedback loop is essential for improving the software.
- Example: Include a “Report a Bug” feature in your task management app, where users can submit bug reports directly from the application.
- Test Documentation:
- Properly document all test cases, including expected results, actual results, and any issues found. This documentation is essential for tracking the testing process and addressing problems.
- Test Reports and Summary:
- Compile test reports summarizing the outcomes of all tests, highlighting issues found, and providing an overall assessment of the software’s readiness for release.
- By following these testing steps, you can thoroughly evaluate your software, identify issues, and ensure that it meets quality standards before deployment.
- Unit Testing:
- Automation:
- Use automated testing tools and continuous integration (CI) pipelines to run tests automatically. This helps catch bugs early and ensures code quality.
- Documentation:
- Document your code and the software’s architecture. This includes inline comments, README files, and user manuals.
- Performance Testing:
- Test the software’s performance under different conditions. Identify and address bottlenecks.
- Security Testing:
- Perform security testing to identify vulnerabilities and address them. This includes code scans and penetration testing.
- Code Refactoring:
- Regularly review your codebase for potential improvements. Refactor code to make it more maintainable and efficient.
- Dependency Management:
- Keep track of and update third-party libraries and dependencies to ensure your software is up to date and secure.
- Bug Tracking:
- Use a bug tracking system to log and manage issues. Prioritize and fix bugs promptly.
- Peer Collaboration:
- Foster a collaborative environment where team members can communicate and share knowledge. This helps improve code quality and problem-solving.
- Code Metrics:
- Use code analysis tools to measure code quality and maintainability. Metrics like cyclomatic complexity and code coverage can help you assess your codebase.
- User Feedback:
- Collect feedback from users and stakeholders to continuously improve the software.
- Release Management:
- Plan and manage software releases. Ensure that each release is thoroughly tested and documented.
- Continuous Improvement:
- Encourage a culture of continuous improvement. Learn from past mistakes and successes, and adapt your processes accordingly.
- Monitoring and Maintenance:
- After deployment, monitor the software in production. Address issues promptly and release updates as needed.
- Training and Support:
- Provide training to end-users and maintain a support system to help them with any issues or questions.
By following these steps and continually focusing on quality, you can ensure that your software code is of high quality and that your software development process is efficient and effective.