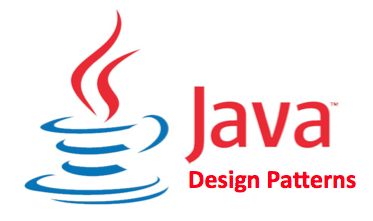
Introduction
Design Patterns are very popular among software developers. A design pattern is a well described solution to a common software problem.
Some of the benefits of using design patterns are:
- Design Patterns are already defined and provides industry standard approach to solve a recurring problem, so it saves time if we sensibly use the design pattern. There are many java design patterns that we can use in our java based projects.
- Using design patterns promotes re-usability that leads to more robust and highly maintainable code. It helps in reducing total cost of ownership (TCO) of the software product.
- Since design patterns are already defined, it makes our code easy to understand and debug. It leads to faster development and new members of team understand it easily.
Java Design Patterns are divided three categories like –
- Creational Design Patterns
- Strutural Design Patterns
- Behavioural Design Patterns
Creational Design Patterns
In software engineering, creational design patterns are design patterns that deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. The basic form of object creation could result in design problems or added complexity to the design.
Types of creational design patterns
There are following 6 types of creational design patterns.
- Factory Method Pattern
- Abstract Factory Pattern
- Singleton Pattern
- Prototype Pattern
- Builder Pattern
- Object Pool Pattern
Strutural Design Patterns
In Software Engineering, Structural Design Patterns are Design Patterns that ease the design by identifying a simple way to realize relationships between entities.
Types of structural design patterns
There are following 7 types of structural design patterns.
- Adapter Pattern
- Bridge Pattern
- Composite Pattern
- Decorator Pattern
- Facade Pattern
- Flyweight Pattern
- proxy Pattern
Behavioural Design Patterns
Behavioral patterns are those which are concerned with interactions between the objects. The interactions between the objects should be such that they are talking to each other and still are loosely coupled. The loose coupling is the key to n-tier architectures. In this, the implementation and the client should be loosely coupled in order to avoid hard-coding and dependencies.
The behavioral patterns are:
- Chain of Responsibility Pattern
- Command Pattern
- Interpreter Pattern
- Iterator Pattern
- Mediator Pattern
- Memento Pattern
- Observer Pattern
- State Pattern
- Strategy Pattern
- Template Pattern
- Visitor Pattern
- Null Object
At any given moment, somewhere in the world someone struggles with the same software design problems you have. You know you don’t want to reinvent the wheel (or worse, a flat tire), so you look to Design Patterns–the lessons learned by those who’ve faced the same problems. With Design Patterns, you get to take advantage of the best practices and experience of others, so that you can spend your time on…something else. Something more challenging. Something more complex. Something more fun.
You want to learn about the patterns that matter–why to use them, when to use them, how to use them (and when NOT to use them). But you don’t just want to see how patterns look in a book, you want to know how they look “in the wild”. In their native environment. In other words, in real world applications. You also want to learn how patterns are used in the Java API, and how to exploit Java’s built-in pattern support in your own code.
You want to learn the real OO design principles and why everything your boss told you about inheritance might be wrong (and what to do instead). You want to learn how those principles will help the next time you’re up a creek without a design pattern.
Most importantly, you want to learn the “secret language” of Design Patterns so that you can hold your own with your co-worker (and impress cocktail party guests) when he casually mentions his stunningly clever use of Command, Facade, Proxy, and Factory in between sips of a martini. You’ll easily counter with your deep understanding of why Singleton isn’t as simple as it sounds, how the Factory is so often misunderstood, or on the real relationship between Decorator, Facade and Adapter.
Christopher Alexander was the first person who invented all the above Design Patterns in 1977.
But later the Gang of Four – Design patterns, elements of reusable object-oriented software book was written by a group of four persons named as Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides in 1995.
That’s why all the above 23 Design Patterns are known as Gang of Four (GoF) Design Patterns.