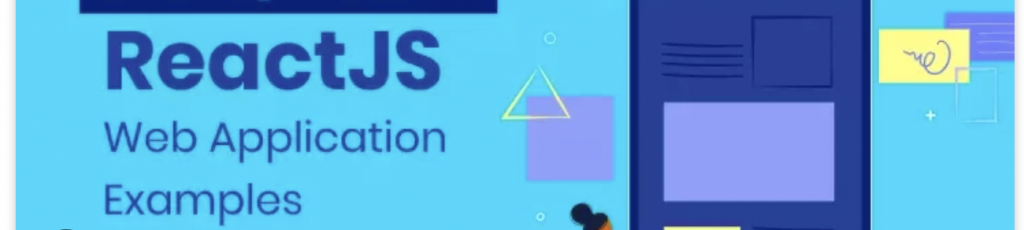
Here’s a sample project that demonstrates how to display employee data using React:
First, make sure you have Node.js and npm (Node Package Manager) installed on your machine. Then, follow these steps:
- Set up a new React project by running the following command in your terminal:
npx create-react-app employee-data-display
Change into the project directory:
cd employee-data-display
cd employee-data-display
- Open the project in your preferred code editor.
- Create a new file called
employees.js
in thesrc
directory and add some sample employee data:
export const employees = [
{
id: 1,
name: "John Doe",
position: "Manager",
department: "Sales",
},
{
id: 2,
name: "Jane Smith",
position: "Developer",
department: "Engineering",
},
{
id: 3,
name: "Bob Johnson",
position: "Designer",
department: "Marketing",
},
];
Replace the contents of src/App.js
with the following code:
import React from 'react';
import { employees } from './employees';
function App() {
return (
<div>
<h1>Employee Data</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Position</th>
<th>Department</th>
</tr>
</thead>
<tbody>
{employees.map((employee) => (
<tr key={employee.id}>
<td>{employee.id}</td>
<td>{employee.name}</td>
<td>{employee.position}</td>
<td>{employee.department}</td>
</tr>
))}
</tbody>
</table>
</div>
);
}
export default App;
Save the changes and run the project using the following command:
npm start
- Open your browser and visit
http://localhost:3000
to see the employee data displayed in a table.
That’s it! You’ve created a basic React application that displays employee data. The sample data is imported from employees.js
, and then it is mapped over to render each employee’s information in a table.
Feel free to modify the code to fit your specific needs and style the table using CSS.