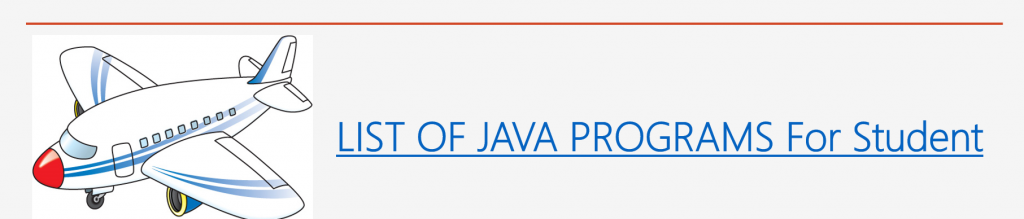
Define a class to accept 10 integers and arrange them in descending order using bubble sort technique print the original array and sorted array.
Here’s a Java program that defines a class to accept 10 integers and arranges them in descending order using the bubble sort technique. It then prints the original array and the sorted array:
import java.util.Scanner;
public class BubbleSortDescending {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Accept 10 integers
int[] array = new int[10];
System.out.println("Enter 10 integers:");
for (int i = 0; i < array.length; i++) {
System.out.print("Enter integer " + (i + 1) + ": ");
array[i] = scanner.nextInt();
}
// Perform bubble sort in descending order
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - 1 - i; j++) {
if (array[j] < array[j + 1]) {
// Swap elements if they are in the wrong order
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
// Print the original array
System.out.print("\nOriginal Array: ");
for (int num : array) {
System.out.print(num + " ");
}
// Print the sorted array
System.out.print("\nSorted Array (Descending): ");
for (int num : array) {
System.out.print(num + " ");
}
scanner.close();
}
}
Define a class to accept 10 character and arrange them in ascending order using bubble sort technique print the original array and sorted array.
Here’s a Java program that defines a class to accept 10 characters and arranges them in ascending order using the bubble sort technique. It then prints the original array and the sorted array:
import java.util.Scanner;
public class BubbleSortChar {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Accept 10 characters
char[] charArray = new char[10];
System.out.println("Enter 10 characters:");
for (int i = 0; i < 10; i++) {
System.out.print("Enter character " + (i + 1) + ": ");
charArray[i] = scanner.next().charAt(0);
}
// Perform bubble sort
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9 - i; j++) {
if (charArray[j] > charArray[j + 1]) {
// Swap characters
char temp = charArray[j];
charArray[j] = charArray[j + 1];
charArray[j + 1] = temp;
}
}
}
// Print original array
System.out.print("\nOriginal array: ");
for (char ch : charArray) {
System.out.print(ch + " ");
}
// Print sorted array
System.out.print("\nSorted array: ");
for (char ch : charArray) {
System.out.print(ch + " ");
}
scanner.close();
}
}
Java Program to Count the Number of Alphabets, Numbers, and Special Characters in a Given String.
Below are two Java programs. The first one calculates the number of alphabets, numbers, and special characters in a given string. The second program takes input from the user:
import java.util.Scanner;
public class CharacterCountUserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a string: ");
String inputString = scanner.nextLine();
int alphabetCount = 0;
int numberCount = 0;
int specialCharCount = 0;
for (char ch : inputString.toCharArray()) {
if (Character.isLetter(ch)) {
alphabetCount++;
} else if (Character.isDigit(ch)) {
numberCount++;
} else {
specialCharCount++;
}
}
System.out.println("Alphabets: " + alphabetCount);
System.out.println("Numbers: " + numberCount);
System.out.println("Special Characters: " + specialCharCount);
scanner.close();
}
}
Write a java program which creates class Student (Rollno, Name,- Number of subjects,Marks of each subject)(Number of subjects varies for each student) Write a parameterized constructor which initializes roll no, name & Number of subjects and create the array of marks dynamically. Display the details of all students with percentage and class obtained.
Below is a Java program that fulfills the given requirements. The program defines a Student
class with a parameterized constructor, dynamically creates an array of marks, and displays details including percentage and class obtained for each student.
import java.util.Scanner;
class Student {
private int rollNo;
private String name;
private int numSubjects;
private int[] marks;
// Parameterized constructor to initialize roll no, name, and number of subjects
public Student(int rollNo, String name, int numSubjects) {
this.rollNo = rollNo;
this.name = name;
this.numSubjects = numSubjects;
// Dynamically create an array of marks based on the number of subjects
this.marks = new int[numSubjects];
}
// Method to input marks for each subject
public void inputMarks(Scanner scanner) {
System.out.println("Enter marks for each subject for student " + rollNo + ":");
for (int i = 0; i < numSubjects; i++) {
System.out.print("Enter marks for Subject " + (i + 1) + ": ");
marks[i] = scanner.nextInt();
}
}
// Method to calculate percentage
public double calculatePercentage() {
int totalMarks = 0;
for (int mark : marks) {
totalMarks += mark;
}
return (double) totalMarks / numSubjects;
}
// Method to determine class obtained based on percentage
public String determineClass() {
double percentage = calculatePercentage();
if (percentage >= 80) {
return "Distinction";
} else if (percentage >= 60) {
return "First Class";
} else if (percentage >= 40) {
return "Pass Class";
} else {
return "Fail";
}
}
// Method to display student details
public void displayDetails() {
System.out.println("\nStudent Details:");
System.out.println("Roll No: " + rollNo);
System.out.println("Name: " + name);
System.out.println("Number of Subjects: " + numSubjects);
System.out.println("Marks: " + java.util.Arrays.toString(marks));
System.out.println("Percentage: " + calculatePercentage() + "%");
System.out.println("Class Obtained: " + determineClass());
}
}
public class StudentDetailsProgram {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input the number of students
System.out.print("Enter the number of students: ");
int numStudents = scanner.nextInt();
// Create an array of Student objects
Student[] students = new Student[numStudents];
// Input details for each student
for (int i = 0; i < numStudents; i++) {
System.out.println("\nEnter details for Student " + (i + 1) + ":");
System.out.print("Enter Roll No: ");
int rollNo = scanner.nextInt();
System.out.print("Enter Name: ");
String name = scanner.next();
System.out.print("Enter Number of Subjects: ");
int numSubjects = scanner.nextInt();
// Create a Student object with input details
students[i] = new Student(rollNo, name, numSubjects);
// Input marks for each subject
students[i].inputMarks(scanner);
}
// Display details for each student
for (Student student : students) {
student.displayDetails();
}
scanner.close();
}
}