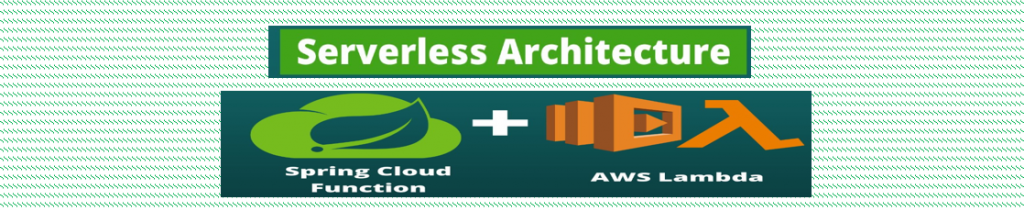
Introduction
We can provide you with an example of a Serverless architecture using Spring Boot. In this example, we’ll create a simple RESTful API using Spring Boot and deploy it to AWS Lambda using the Serverless Framework.
Here are the steps to create a Serverless Spring Boot project:
Step 1: Set up the Development Environment
- Install Java JDK and Maven.
- Install Node.js and npm.
- Install the Serverless Framework by running the command:
npm install -g serverless
Step 2: Create a new Spring Boot Project
- Open your terminal and navigate to the directory where you want to create the project.
- Run the following command to create a new Spring Boot project using Spring Initializr:
curl https://start.spring.io/starter.zip \
-d dependencies=web \
-d language=java \
-d javaVersion=11 \
-d type=maven-project \
-d groupId=com.example \
-d artifactId=myserverlessapp \
-d packageName=com.example.myserverlessapp \
-o myserverlessapp.zip
- Unzip the generated file using the command:
unzip myserverlessapp.zip
- Navigate into the project directory:
cd myserverlessapp
Step 3: Implement the RESTful API
- Open the
src/main/java/com/example/myserverlessapp/MyServerlessAppApplication.java
file and add the@SpringBootApplication
annotation. - Create a new Java class
HelloController.java
in thesrc/main/java/com/example/myserverlessapp/controller
directory with the following code:
package com.example.myserverlessapp.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, Serverless World!";
}
}
Step 4: Build the Application
- Open your terminal and navigate to the project root directory (
myserverlessapp
). - Run the following command to build the project:
mvn clean package
Step 5: Configure the Serverless Framework
- Create a new file
serverless.yml
in the project root directory with the following contents:
service: my-serverless-app
provider:
name: aws
runtime: java11
stage: dev
region: us-east-1
functions:
hello:
handler: org.springframework.cloud.function.adapter.aws.FunctionInvoker::handleRequest
events:
- http:
path: hello
method: get
- Save the
serverless.yml
file.
Step 6: Deploy the Application to AWS Lambda
- Open your terminal and navigate to the project root directory (
myserverlessapp
). - Run the following command to deploy the application:
serverless deploy
After the deployment is complete, you will receive an endpoint URL that you can use to access your API.
That’s it! You have created a Serverless architecture using Spring Boot and deployed it to AWS Lambda using the Serverless Framework. You can now test your API by sending a GET request to the provided endpoint URL followed by /api/hello
. It should return the message “Hello, Serverless World!”.