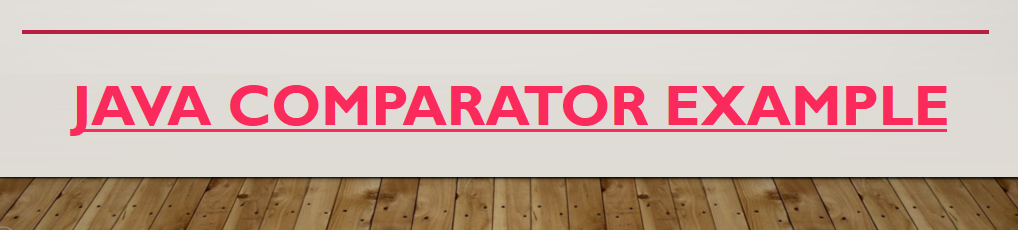
Introduction
The Java Comparator class helps to understand how a comparator implemented in Java programming language. This program has Reporter class which and the object of this class will be used to sort by age.
Please find the entire program below –
Java Program Code
KWComparatorExample.java
This is the main class which start execution of the program.
package com.kw.sample;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* This class helps to demonstrate the implementation and usages of Comparator.
*
* @author dsahu1
*
*/
public class KWComparatorExample {
/**
* This is the main method which start execution of the program.
*
* @param args
*/
public static void main(String args[]) {
Map<String, KWReporter> reporter = new HashMap<String, KWReporter>();
KWReporter jim = new KWReporter("Jonny", "Smith", null, 25);
KWReporter scott = new KWReporter("Tiger", "Scott", null, 28);
KWReporter anna = new KWReporter("Bonny", "Kapoor", null, 23);
KWReporter david = new KWReporter("Mike", "Roy", null, 06);
KWReporter john = new KWReporter("john", "Thomas", null, 55);
reporter.put(jim.getFirstName(), jim);
reporter.put(scott.getFirstName(), scott);
reporter.put(anna.getFirstName(), anna);
reporter.put(david.getFirstName(), david);
reporter.put(john.getFirstName(), john);
// not yet sorted
List<KWReporter> reporterByAge = new ArrayList<KWReporter>(reporter.values());
// Call API to sort the list
Collections.sort(reporterByAge, new Comparator<KWReporter>() {
public int compare(KWReporter o1, KWReporter o2) {
return o1.getAge() - o2.getAge();
}
});
// Iterate the List and it will be sort by age
for (KWReporter reporter1 : reporterByAge) {
System.out.println(reporter1.getFirstName() + "\t"
+ reporter1.getFirstName() + "\t"
+ reporter1.getAge());
}
}
}
KWReporter.java
This is an POJO class which helps to hold the data.
package com.kw.sample;
import java.util.Date;
/**
* This class helps to contains reporter information like first name, last name,
* dob and age.
*
* @author dsahu1
*
*/
public class KWReporter {
// Attribute to hold first Name
private String firstName;
// Attribute to hold Last Name
private String lastName;
// Attribute to hold Data Of Birth
private Date dateOfBirth;
// Attribute to hold age
private int age;
// Constructor to instantiate object
public KWReporter(String firstName, String lastName, Date dateOfBirth,
int age) {
this.firstName = firstName;
this.lastName = lastName;
this.dateOfBirth = dateOfBirth;
this.age = age;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public Date getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(Date dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Output of the Program
- The user can execute this program using the eclipse. Right Click on the program KWComparatorExample .java and click on Run – > Java Application