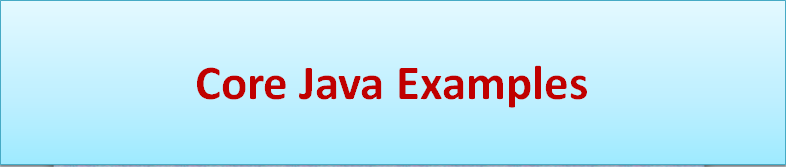
Average of three number
import java.util.Scanner;
public class AverageCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input: Getting three numbers from the user
System.out.print("Enter the first number: ");
double num1 = scanner.nextDouble();
System.out.print("Enter the second number: ");
double num2 = scanner.nextDouble();
System.out.print("Enter the third number: ");
double num3 = scanner.nextDouble();
// Processing: Calculating the average
double average = calculateAverage(num1, num2, num3);
// Output: Displaying the result
System.out.println("The average of the three numbers is: " + average);
// Closing the scanner to prevent resource leak
scanner.close();
}
// Function to calculate the average
private static double calculateAverage(double num1, double num2, double num3) {
return (num1 + num2 + num3) / 3;
}
}
Calculate Area of a Circle
import java.util.Scanner;
public class CircleArea {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the radius of the circle: ");
double radius = scanner.nextDouble();
double area = Math.PI * Math.pow(radius, 2);
System.out.println("The area of the circle is: " + area);
scanner.close();
}
}
Factorial Calculation
import java.util.Scanner;
public class Factorial {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
long factorial = 1;
for (int i = 1; i <= num; ++i) {
factorial *= i;
}
System.out.println("The factorial of " + num + " is: " + factorial);
scanner.close();
}
}
Check if a Number is Prime
import java.util.Scanner;
public class PrimeCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
boolean isPrime = true;
for (int i = 2; i <= num / 2; ++i) {
if (num % i == 0) {
isPrime = false;
break;
}
}
System.out.println(num + (isPrime ? " is" : " is not") + " a prime number.");
scanner.close();
}
}
Fibonacci Series
import java.util.Scanner;
public class Fibonacci {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the number of terms: ");
int n = scanner.nextInt();
int firstTerm = 0, secondTerm = 1;
System.out.println("Fibonacci Series:");
for (int i = 1; i <= n; ++i) {
System.out.print(firstTerm + ", ");
int nextTerm = firstTerm + secondTerm;
firstTerm = secondTerm;
secondTerm = nextTerm;
}
scanner.close();
}
}
Palindrome Check
import java.util.Scanner;
public class PalindromeCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
int originalNum = num;
int reversedNum = 0;
while (num != 0) {
int digit = num % 10;
reversedNum = reversedNum * 10 + digit;
num /= 10;
}
System.out.println(originalNum + (originalNum == reversedNum ? " is" : " is not") + " a palindrome.");
scanner.close();
}
}
Sum of Digits
import java.util.Scanner;
public class SumOfDigits {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
int sum = 0;
while (num != 0) {
int digit = num % 10;
sum += digit;
num /= 10;
}
System.out.println("The sum of digits is: " + sum);
scanner.close();
}
}
Matrix Addition
import java.util.Scanner;
public class MatrixAddition {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int rows, columns;
System.out.print("Enter the number of rows: ");
rows = scanner.nextInt();
System.out.print("Enter the number of columns: ");
columns = scanner.nextInt();
int[][] matrixA = new int[rows][columns];
int[][] matrixB = new int[rows][columns];
int[][] resultMatrix = new int[rows][columns];
// Input matrices
System.out.println("Enter elements of matrix A:");
inputMatrix(matrixA, scanner);
System.out.println("Enter elements of matrix B:");
inputMatrix(matrixB, scanner);
// Perform matrix addition
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < columns; ++j) {
resultMatrix[i][j] = matrixA[i][j] + matrixB[i][j];
}
}
// Display result
System.out.println("Resultant matrix after addition:");
displayMatrix(resultMatrix);
scanner.close();
}
private static void inputMatrix(int[][] matrix, Scanner scanner) {
for (int i = 0; i < matrix.length; ++i) {
for (int j = 0; j < matrix[0].length; ++j) {
System.out.print("Enter element at position " + (i + 1) + "," + (j + 1) + ": ");
matrix[i][j] = scanner.nextInt();
}
}
}
private static void displayMatrix(int[][] matrix) {
for (int[] row : matrix) {
for (int element : row) {
System.out.print(element + " ");
}
System.out.println();
}
}
}
Reverse a String
import java.util.Scanner;
public class ReverseString {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a string: ");
String input = scanner.nextLine();
String reversed = reverseString(input);
System.out.println("Reversed string: " + reversed);
scanner.close();
}
private static String reverseString(String str) {
StringBuilder reversed = new StringBuilder();
for (int i = str.length() - 1; i >= 0; i--) {
reversed.append(str.charAt(i));
}
return reversed.toString();
}
}
Armstrong Number Check
import java.util.Scanner;
public class ArmstrongNumberCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
boolean isArmstrong = checkArmstrong(num);
System.out.println(num + (isArmstrong ? " is" : " is not") + " an Armstrong number.");
scanner.close();
}
private static boolean checkArmstrong(int num) {
int originalNum = num;
int digits = (int) Math.log10(num) + 1;
int sum = 0;
while (num > 0) {
int digit = num % 10;
sum += Math.pow(digit, digits);
num /= 10;
}
return sum == originalNum;
}
}
Binary to Decimal Conversion
import java.util.Scanner;
public class BinaryToDecimal {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a binary number: ");
long binaryNum = scanner.nextLong();
int decimalNum = convertBinaryToDecimal(binaryNum);
System.out.println("Decimal equivalent: " + decimalNum);
scanner.close();
}
private static int convertBinaryToDecimal(long binaryNum) {
int decimalNum = 0;
int power = 0;
while (binaryNum != 0) {
int digit = (int) (binaryNum % 10);
decimalNum += digit * Math.pow(2, power);
power++;
binaryNum /= 10;
}
return decimalNum;
}
}
Factorial using Recursion
import java.util.Scanner;
public class FactorialRecursion {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
long factorial = calculateFactorial(num);
System.out.println("The factorial of " + num + " is: " + factorial);
scanner.close();
}
private static long calculateFactorial(int num) {
if (num == 0 || num == 1) {
return 1;
} else {
return num * calculateFactorial(num - 1);
}
}
}
Check Leap Year
import java.util.Scanner;
public class LeapYearCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a year: ");
int year = scanner.nextInt();
boolean isLeapYear = checkLeapYear(year);
System.out.println(year + (isLeapYear ? " is" : " is not") + " a leap year.");
scanner.close();
}
private static boolean checkLeapYear(int year) {
// A year is a leap year if it is divisible by 4 but not divisible by 100,
// or it is divisible by 400.
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
}
Write a program to enter two numbers and check whether they are co-prime or not.
Below is a Java program that takes two numbers as input and checks whether they are co-prime or not. Two numbers are considered co-prime if their greatest common divisor (GCD) is 1.
import java.util.Scanner;
public class CoPrimeChecker {
// Method to calculate the GCD of two numbers using Euclidean Algorithm
public static int calculateGCD(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input two numbers
System.out.print("Enter the first number: ");
int num1 = scanner.nextInt();
System.out.print("Enter the second number: ");
int num2 = scanner.nextInt();
// Check if the numbers are co-prime
if (calculateGCD(num1, num2) == 1) {
System.out.println(num1 + " and " + num2 + " are co-prime numbers.");
} else {
System.out.println(num1 + " and " + num2 + " are not co-prime numbers.");
}
scanner.close();
}
}
Explanation:
The program uses a method calculateGCD to find the greatest common divisor (GCD) of two numbers using the Euclidean Algorithm.
The main method takes two numbers as input from the user.
It then calls the calculateGCD method to find the GCD of the two numbers.
If the GCD is 1, the numbers are considered co-prime, and a message is displayed indicating that. Otherwise, a message is displayed indicating that the numbers are not co-prime.
The Scanner is closed to prevent resource leaks.
This program demonstrates a simple approach to check whether two numbers are co-prime or not.
Write a program to display all the ‘Buzz Numbers’ between p and q (where p<q). A ‘Buzz Number’ is the number which ends with 7 or is divisible by 7.
Below is a Java program that displays all the “Buzz Numbers” between p
and q
(where p
is less than q
). A “Buzz Number” is defined as a number that either ends with 7 or is divisible by 7.
import java.util.Scanner;
public class BuzzNumbers {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input values for p and q
System.out.print("Enter the value of p: ");
int p = scanner.nextInt();
System.out.print("Enter the value of q (q should be greater than p): ");
int q = scanner.nextInt();
// Validate input
if (p >= q) {
System.out.println("Invalid input. q should be greater than p.");
return;
}
System.out.println("Buzz Numbers between " + p + " and " + q + ":");
displayBuzzNumbers(p, q);
scanner.close();
}
// Method to check if a number is a Buzz Number
private static boolean isBuzzNumber(int num) {
return num % 10 == 7 || num % 7 == 0;
}
// Method to display Buzz Numbers between p and q
private static void displayBuzzNumbers(int p, int q) {
for (int i = p; i <= q; i++) {
if (isBuzzNumber(i)) {
System.out.print(i + " ");
}
}
}
}
Explanation:
The program starts by taking input values for p and q from the user.
It then validates the input to ensure that q is greater than p. If not, it prints an error message and terminates the program.
The isBuzzNumber method checks if a given number is a Buzz Number according to the definition provided (ends with 7 or is divisible by 7).
The displayBuzzNumbers method iterates through the numbers from p to q and prints the Buzz Numbers.
The main method calls these methods to execute the program. It prompts the user for input, validates it, and then displays the Buzz Numbers within the specified range.