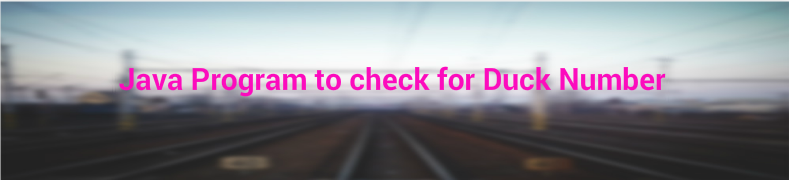
Introduction
This program helps to check weather number is a Duck Number or not.
A Duck number is a number which has zeroes present in it, but there should be no zero present in the beginning of the number.
For example 3210, 7056, 8430709 are all duck numbers whereas 08237, 04309 are not.
Explanation:
- Number 3210 is having zero at 4th place and first digit is not zero so it is Duck Number.
- Number 08237 is having zero at first place but not in other place so it is not a Duck Number.
- Number 04309 is not a Duck Number because it is having first Digit as a Zero and it indicate it. It does not matter anywhere else to have Zero, if first digit is zero.
Flowchart
Please find sample flowchart diagram for this program as given below –
Program (Code)
This Number helps to check duck number, please find java program below –
package com.kw.sample;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
/**
* This class helps to check weather number is a duck number or not.
*
* @author dsahu1
*
*/
public class KWDuckNumberProgram {
/**
* This method helps to validate number as duck number or not.
*
* @param args
* @throws IOException
*/
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter any number : ");
String num = br.readLine();
int length = num.length();
int count = 0;
char ch;
for (int i = 1; i < length; i++) {
ch = num.charAt(i);
if (ch == '0')
count++;
}
char f = num.charAt(0);
if (count > 0 && f != '0')
System.out.println(num + " is a duck number");
else
System.out.println(num + " is not a duck number");
}
}
Output
Once execute above program then we will be able to get below output by passing number.
Scenario1:
Enter any number : 256001
256001 is a duck number
Scenario2:
Enter any number : 0235401
0235401 is not a duck number
Video Explanation
This video helps to understand flowchart and program which written in the above steps. There are various ways to write a program and we are not covering all of them as this is just for practice. Please post your program in the comment box so other can utilize it.