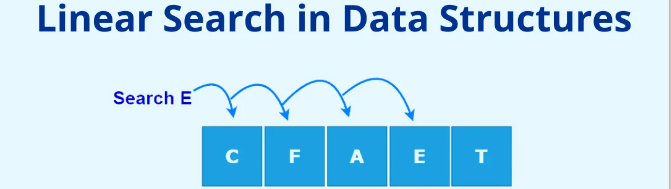
Overview
Linear search is a simple searching algorithm that iterates through the elements of a list or array in a linear manner to find a specific value. It is straightforward but may not be the most efficient for large datasets.
Code
import java.util.Scanner;
public class LinearSearch {
public static int linearSearch(int[] array, int key) {
for (int i = 0; i < array.length; i++) {
if (array[i] == key) {
return i; // Return the index of the key if found
}
}
return -1; // Return -1 if the key is not found
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input: Array Elements
System.out.print("Enter the number of elements in the array: ");
int n = scanner.nextInt();
int[] array = new int[n];
System.out.println("Enter the array elements:");
for (int i = 0; i < n; i++) {
array[i] = scanner.nextInt();
}
// Input: Element to be searched
System.out.print("Enter the element to be searched: ");
int key = scanner.nextInt();
// Perform Linear Search
int index = linearSearch(array, key);
// Output: Display result
if (index != -1) {
System.out.println("Element found at index " + index);
} else {
System.out.println("Element not found in the array");
}
}
}
Explanation:
The linearSearch
method takes an array and a key as parameters. It iterates through the array using a for
loop and checks if each element is equal to the key.
If the key is found, the method returns the index of the key. If the key is not found, it returns -1.
In the main
method, the user is prompted to enter the number of elements in the array and the array elements.
The user is then asked to enter the element to be searched (key
).
The linearSearch
method is called, and the result is displayed.This program demonstrates a simple linear search algorithm in Java.
Linear Search in a 2D Array in Java
Linear search can also be applied to a two-dimensional array. In this example, we will search for a specific element in a 2D array.
Java Code
import java.util.Scanner;
public class TwoDArrayLinearSearch {
public static int[] linearSearch2D(int[][] array, int key) {
int rows = array.length;
int cols = array[0].length;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (array[i][j] == key) {
int[] result = { i, j }; // Return the row and column indices if found
return result;
}
}
}
int[] notFound = { -1, -1 }; // Return {-1, -1} if the key is not found
return notFound;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input: 2D Array Elements
System.out.print("Enter the number of rows in the 2D array: ");
int rows = scanner.nextInt();
System.out.print("Enter the number of columns in the 2D array: ");
int cols = scanner.nextInt();
int[][] array = new int[rows][cols];
System.out.println("Enter the 2D array elements:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
array[i][j] = scanner.nextInt();
}
}
// Input: Element to be searched
System.out.print("Enter the element to be searched: ");
int key = scanner.nextInt();
// Perform Linear Search in 2D Array
int[] result = linearSearch2D(array, key);
// Output: Display result
if (result[0] != -1 && result[1] != -1) {
System.out.println("Element found at position (" + result[0] + ", " + result[1] + ")");
} else {
System.out.println("Element not found in the 2D array");
}
}
}
Explanation:
- The
linearSearch2D
method takes a 2D array and a key as parameters. It iterates through the elements of the 2D array using nested loops and checks if each element is equal to the key. - If the key is found, the method returns an array containing the row and column indices. If the key is not found, it returns {-1, -1}.
- In the
main
method, the user is prompted to enter the number of rows and columns in the 2D array and the array elements. - The user is then asked to enter the element to be searched (
key
). - The
linearSearch2D
method is called, and the result is displayed. - This program demonstrates a linear search in a 2D array in Java.