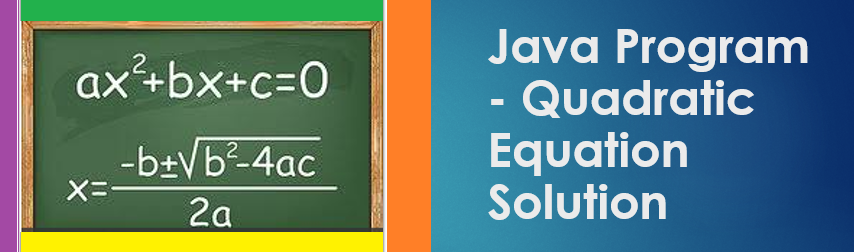
Introduction
This Java Application helps to find out the value of X for a quadratic equation using the Shridharacharya formula.
Sridhar Acharya (c. 870, India – c. 930 India) was an Indian mathematician, Sanskrit pundit andphilosopher. He was born in Bhurishresti (Bhurisristi or Bhurshut) village in South Radha (at present Hughli) in the 10th Century AD.
He was known for two treatises: Trisatika(sometimes called the Patiganitasara) and thePatiganita. His major work Patiganitasara was named Trisatika because it was written in three hundred slokas. The book discusses counting of numbers, measures, natural number, multiplication, division, zero, squares, cubes, fraction, rule of three, interest-calculation, joint business or partnership and mensuration.
He was one of the first to give a formula for solving quadratic equations.He found the formula:
Formula:
Java Program Code
KWQuadraticEquationSolution.java: This is the main class which helps to start the execution of the program.
The main method added in this class. The user can generate the executable jar file through eclipse or command line.
package com.kw.javaswing;
import javax.swing.JFrame;
/**
* This is the main class which start execution of the program. Quadratic
* Equation: (aX^2+bX+c=0)
*
* @author dsahu1
*
*/
public class KWQuadraticEquationSolution {
/**
* This method helps to execute the program.
*
* @param args
*/
public static void main(String[] args) {
JFrame frame = new JFrame("Quadratic Equation Application");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Instantiate Panel
KWQuadraticEquationPanel panel = new KWQuadraticEquationPanel();
// Add Panel to Frame
frame.getContentPane().add(panel);
frame.pack();
frame.setVisible(true);
}
}
KWQuadraticEquationPanel.java: This class helps to construct the UI to display the quadratic equation input text field and calculate button along with result area.
package com.kw.javaswing;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.border.TitledBorder;
/**
* This class helps to generate Panel specific to the program.
*
* @author dsahu1
*
*/
public class KWQuadraticEquationPanel extends JPanel {
private static final long serialVersionUID = 1000000L;
// Attribute to hold label for the UI
private JLabel inputLabel, outputLabel, resultLabel, srx, x;
// Attribute to hold Text Field for coefficient a
private JTextField aTextField;
// Attribute to hold Text Field for coefficient b
private JTextField bTextField;
// Attribute to hold Text Field for coefficient c
private JTextField cTextField;
// Attribute to hold button to calculate the value of X
private JButton calculateButton;
/**
* This constructor helps to generate the UI.
*/
public KWQuadraticEquationPanel() {
// Set the Border for main Panel
TitledBorder mainBorder = new TitledBorder(
"Quadratic Equation Application");
mainBorder.setTitleJustification(TitledBorder.CENTER);
mainBorder.setTitlePosition(TitledBorder.TOP);
setBorder(mainBorder);
// Instantiate Labels and Text Button
inputLabel = new JLabel(
"Enter Quadratic Equation(aX^2+bX+c) Coeficiant Value(s):");
outputLabel = new JLabel("Value(s) of X: ");
resultLabel = new JLabel("---");
srx = new JLabel("X^2");
x = new JLabel("X");
aTextField = new JTextField(2);
bTextField = new JTextField(2);
cTextField = new JTextField(2);
calculateButton = new JButton("Calculate");
// Add all other component like Label, Text Field and Button to the
// Panel
JPanel subPanel1 = new JPanel();
subPanel1.setBounds(0, 0, 250, 100);
// subPanel1.setPreferredSize(new Dimension(150, 200));
subPanel1.setBackground(Color.green);
subPanel1.add(inputLabel);
subPanel1.add(aTextField);
subPanel1.add(srx);
subPanel1.add(bTextField);
subPanel1.add(x);
subPanel1.add(cTextField);
// Set up second subpanel
JPanel subPanel2 = new JPanel();
subPanel2.setBounds(0, 50, 250, 100);
TitledBorder resultBorder = new TitledBorder(
"Quadratic Equation Application");
resultBorder.setTitleJustification(TitledBorder.CENTER);
resultBorder.setTitlePosition(TitledBorder.TOP);
subPanel2.setBackground(Color.white);
subPanel2.setBorder(resultBorder);
// Set up first subpanel
// Set up second subpanel
JPanel subPanel3 = new JPanel();
subPanel3.setBounds(0, 150, 250, 100);
// subPanel2.setPreferredSize(new Dimension(150, 200));
subPanel3.setBackground(Color.red);
subPanel3.add(calculateButton);
subPanel3.add(outputLabel);
subPanel3.add(resultLabel);
add(subPanel1);
// add(subPanel2);
add(subPanel3);
// configure Listener when Clicked on Button
calculateButton.addActionListener(new ConversionListener());
// Set Panel Size
setPreferredSize(new Dimension(500, 220));
// Set Panel Color
setBackground(Color.LIGHT_GRAY.brighter());
}
/**
* This Listener Class helps to calculate the value of X once the user
* clicked on the Calculate button.
*
* @author dsahu1
*
*/
private class ConversionListener implements ActionListener {
public void actionPerformed(ActionEvent event) {
// attribute to hold coefficient a
int a = new Integer(aTextField.getText());
// attribute to hold coefficient b
int b = new Integer(bTextField.getText());
// attribute to hold coefficient c
int c = new Integer(cTextField.getText());
// Instantiate a array
// double[] dArray = null;
List<Double> dList = new ArrayList<Double>();
double d = 0.0;
// Use Formula to calculate the value of X
double det = b * b - 4 * a * c;
StringBuilder result = new StringBuilder("");
if (det >= 0) {
// dArray = new double[2];
d = (-b + Math.sqrt(det)) / (2 * a);
dList.add(new Double(String.format("%.2f", d)));
d = (-b - Math.sqrt(det)) / (2 * a);
dList.add(new Double(String.format("%.2f", d)));
}else{
result.delete(0, result.length());
int detAsInteger = (int) -det;
result.append("(" + b + "+√" + detAsInteger + "i)/" + 2 * a);
result.append(", (" + b + "-√" + detAsInteger + "i)/" + 2 * a);
}
int count = 0;
for (double dTemp : dList) {
result.append(dTemp);
if (count != dList.size() - 1) {
result.append("\t,");
}
count++;
}
// Set the value of X to result Label
resultLabel.setText(result.toString());
}
}
}
Program execution and Output
- The user can execute this program using the eclipse. Right Click on the program KWQuadraticEquationSolution.java and click on Run – > Java Application
- Once Java Program executed then it navigates to below screen.
- The user has to enter the value of a, b and c to complete the quadratic equation. The negative values can be entered using the negative symbol.
- Once the user clicked on the Calculate button then the values of x display on the screen.
- Negative values scenario are given below –